Upload New Dataset Data
This page demonstrates a short program that uses the Quantemplate API to upload a file to update new CSV or XLSX data to a dataset
Prerequisites
Before trying this example, make sure you have followed the guide to Setting Up a Connection
Step 1: Configure sharing in-app
In the Quantemplate app, we navigate to the dataset that we want to update. We share the document with the API User in 'Can Edit' mode.
Make a note of the IDs in the URL. The important parts are the Organisation ID and the Dataset ID. In the Dataset view, the URL is constructed like so:
app.quantemplate.com/organisation id/dataset/dataset id/preview
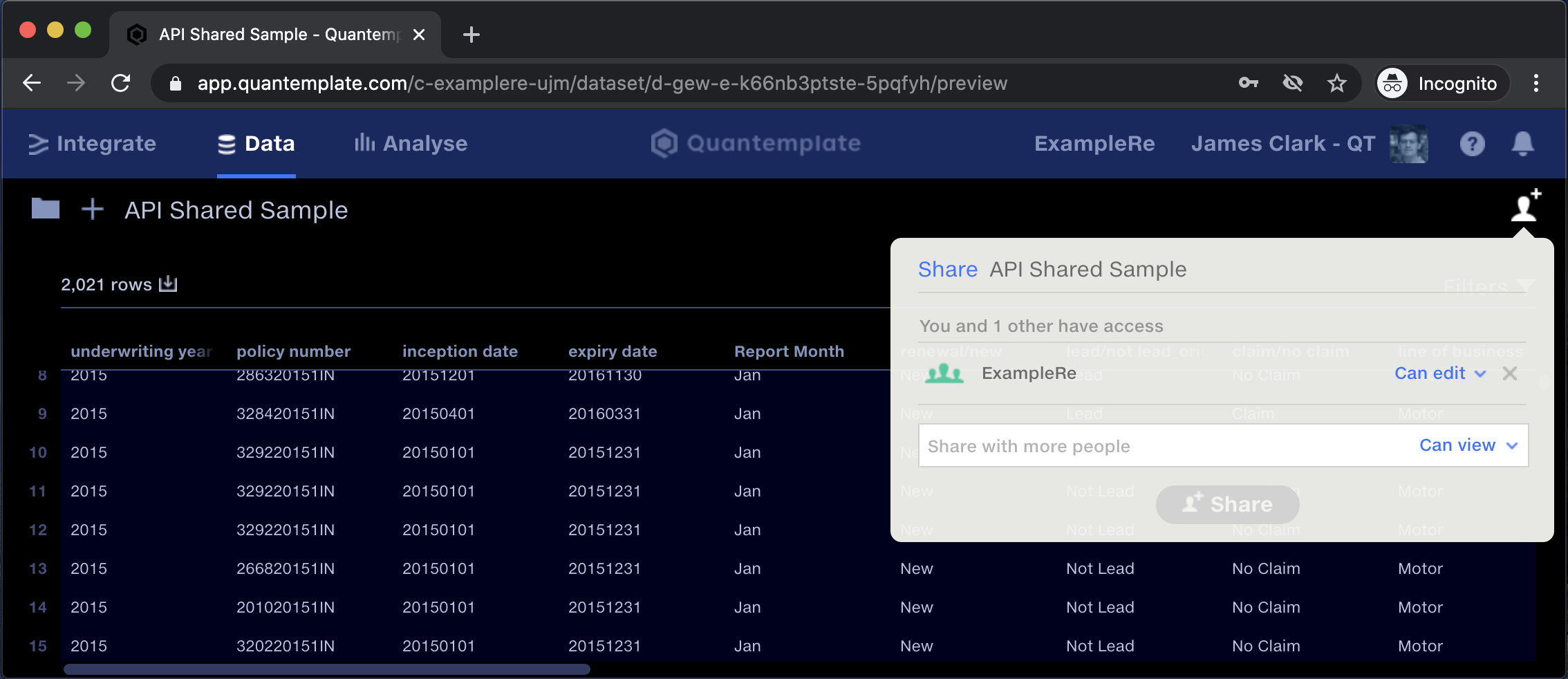
Check the URL to get the relevant IDs, and share the dataset with the whole organisation. 'Can edit' access is required.
In this example:
- Organisation ID is
c-examplere-ujm
- Dataset ID is
d-gew-e-k66nb3ptste-5pqfyh
Step 2: Call the API
Endpoint Limitations
The API documentation uses the URL https://api.prod.quantemplate.com, which has the following limitations:
- file is limited size to 5MB
- Excel files are not supported
The examples on this page use the URL https://fabric.prod.quantemplate.com/external/v1. This has no file size limit, though very large files (gigabytes) are currently untested.
With our Access Token retrieved from the authentication endpoint, we can then use it to make requests to the API.
In this example, we'll be calling the Upload Dataset endpoint. We can see from the API documentation that this URL is parameterised by the Organisation ID and the Dataset ID.
Parameterising the URL with our values above gives us https://fabric.prod.quantemplate.com/external/v1/organisations/c-examplere-ujm/datasets/d-gew-e-k66nb3ptste-5pqfyh
We also need to provide our access token, by setting the Authorization
header to Bearer <token>
Code (Python 3)
The code below demonstrates first authenticating with the API, and then using the access token to upload new dataset data. It requires the requests library.
#!/usr/bin/env python3
import requests
file_to_upload = './ingress.csv' # the CSV or XLSX to upload
user_id = ... # see Setting Up a Connection
secret = ... # see Setting Up a Connection
org_id = ... # your Organisation ID from Step 1
dataset_id = ... # your Dataset ID from Step 1
fabric_endpoint = 'https://fabric.prod.quantemplate.com'
def authenticate(user_id, secret):
return requests.post('https://accounts.prod.quantemplate.com/auth/realms/qt/protocol/openid-connect/token', data = {
'grant_type': 'client_credentials',
'client_id': user_id,
'client_secret': secret
}).json()
def upload(file_path, access_token, org_id, dataset_id):
url = f'{fabric_endpoint}/external/v1/organisations/{org_id}/datasets/{dataset_id}'
with open(file_path, mode='rb') as f:
return requests.post(url, data = f, headers = {
'Authorization': f'Bearer {access_token}'
})
auth_result = authenticate(user_id, secret)
access_token = auth_result['access_token']
res = upload(file_to_upload, access_token, org_id, dataset_id)
# we expect to see a 200 status code if the upload was successful
print(res.status_code)
When we run this program, we should see a '200' printed to console - and when we check the UI, the dataset has been updated with our CSV data.
Updated over 1 year ago